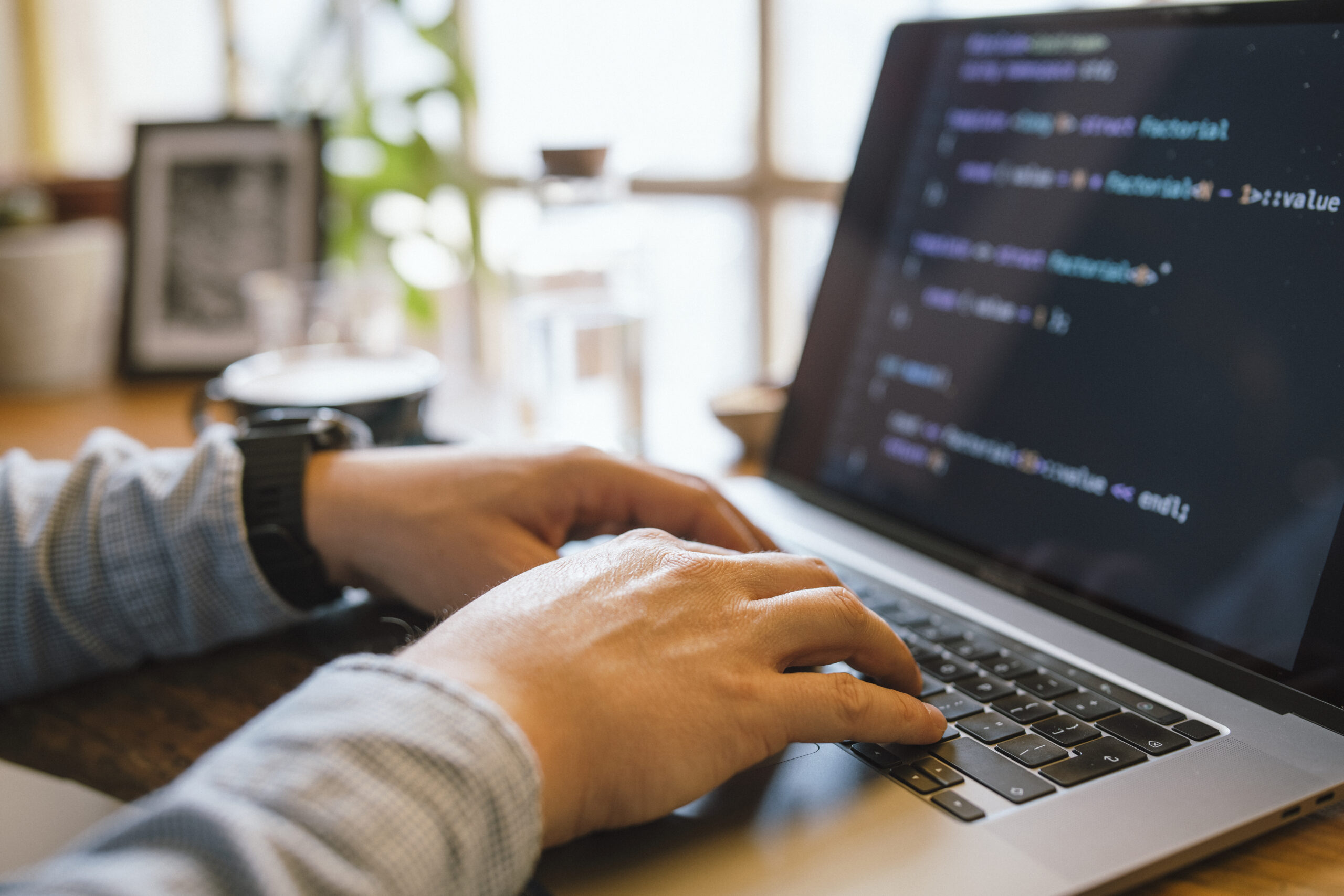
Debugging is The most important — nevertheless generally forgotten — skills inside a developer’s toolkit. It is not almost repairing damaged code; it’s about comprehending how and why items go Mistaken, and Mastering to Assume methodically to unravel problems effectively. No matter whether you are a novice or possibly a seasoned developer, sharpening your debugging techniques can help save hrs of frustration and dramatically improve your efficiency. Here i will discuss quite a few tactics to help developers amount up their debugging video game by me, Gustavo Woltmann.
Grasp Your Equipment
One of many fastest techniques developers can elevate their debugging skills is by mastering the applications they use everyday. While producing code is just one Section of advancement, understanding how to interact with it effectively all through execution is Similarly crucial. Modern enhancement environments arrive equipped with powerful debugging abilities — but several builders only scratch the floor of what these resources can perform.
Choose, by way of example, an Integrated Progress Surroundings (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications assist you to set breakpoints, inspect the worth of variables at runtime, phase via code line by line, and perhaps modify code within the fly. When used correctly, they Enable you to notice specifically how your code behaves during execution, that's a must have for monitoring down elusive bugs.
Browser developer resources, for instance Chrome DevTools, are indispensable for front-conclude developers. They let you inspect the DOM, observe community requests, view true-time performance metrics, and debug JavaScript while in the browser. Mastering the console, resources, and network tabs can transform aggravating UI difficulties into manageable duties.
For backend or process-level developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB give deep Manage about running processes and memory management. Learning these equipment could possibly have a steeper Discovering curve but pays off when debugging overall performance troubles, memory leaks, or segmentation faults.
Outside of your IDE or debugger, become relaxed with Variation control methods like Git to comprehend code record, obtain the precise minute bugs ended up released, and isolate problematic variations.
Ultimately, mastering your tools indicates heading outside of default configurations and shortcuts — it’s about acquiring an personal expertise in your enhancement environment in order that when troubles occur, you’re not dropped in the dead of night. The higher you recognize your instruments, the greater time you could expend resolving the particular challenge in lieu of fumbling by the procedure.
Reproduce the condition
One of the more significant — and infrequently neglected — methods in successful debugging is reproducing the situation. Ahead of jumping into the code or earning guesses, builders need to have to create a regular surroundings or scenario where the bug reliably seems. Without having reproducibility, fixing a bug results in being a game of prospect, generally resulting in wasted time and fragile code variations.
Step one in reproducing an issue is gathering just as much context as you can. Inquire thoughts like: What actions led to The problem? Which setting was it in — improvement, staging, or output? Are there any logs, screenshots, or error messages? The greater depth you have got, the less complicated it gets to isolate the exact problems under which the bug happens.
When you’ve gathered sufficient facts, try and recreate the issue in your neighborhood environment. This may imply inputting the exact same information, simulating comparable person interactions, or mimicking method states. If The problem seems intermittently, contemplate producing automated checks that replicate the edge situations or point out transitions involved. These assessments don't just assist expose the condition but additionally protect against regressions Down the road.
At times, The problem may be surroundings-precise — it might come about only on sure operating programs, browsers, or less than particular configurations. Making use of applications like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms is usually instrumental in replicating such bugs.
Reproducing the trouble isn’t merely a action — it’s a state of mind. It needs endurance, observation, and also a methodical solution. But once you can regularly recreate the bug, you're by now midway to correcting it. That has a reproducible state of affairs, You may use your debugging tools more effectively, take a look at probable fixes safely and securely, and converse extra Evidently with all your workforce or buyers. It turns an abstract complaint right into a concrete obstacle — Which’s in which developers thrive.
Read and Realize the Error Messages
Error messages will often be the most beneficial clues a developer has when a little something goes Completely wrong. In lieu of looking at them as disheartening interruptions, builders must discover to treat mistake messages as direct communications in the method. They often show you just what exactly took place, in which it happened, and in some cases even why it took place — if you know the way to interpret them.
Commence by studying the information meticulously and in full. Quite a few developers, particularly when under time force, glance at the main line and quickly begin earning assumptions. But deeper inside the mistake stack or logs may possibly lie the accurate root bring about. Don’t just copy and paste mistake messages into engines like google — read and fully grasp them initially.
Break the mistake down into components. Can it be a syntax error, a runtime exception, or maybe a logic error? Does it stage to a certain file and line quantity? What module or purpose triggered it? These inquiries can guide your investigation and position you towards the dependable code.
It’s also helpful to grasp the terminology of the programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java often comply with predictable styles, and Finding out to acknowledge these can significantly hasten your debugging process.
Some mistakes are obscure or generic, As well as in those situations, it’s essential to examine the context where the error occurred. Check out similar log entries, input values, and recent changes inside the codebase.
Don’t forget compiler or linter warnings possibly. These often precede greater troubles and supply hints about opportunity bugs.
Ultimately, mistake messages are usually not your enemies—they’re your guides. Learning to interpret them properly turns chaos into clarity, aiding you pinpoint difficulties speedier, cut down debugging time, and turn into a a lot more productive and self-confident developer.
Use Logging Correctly
Logging is Among the most impressive applications in a developer’s debugging toolkit. When used successfully, it provides actual-time insights into how an application behaves, supporting you recognize what’s occurring beneath the hood with no need to pause execution or stage with the code line by line.
A great logging technique starts with understanding what to log and at what level. Common logging concentrations involve DEBUG, Facts, Alert, Mistake, and Deadly. Use DEBUG for thorough diagnostic details in the course of improvement, INFO for general situations (like prosperous start-ups), Alert for likely concerns that don’t break the applying, Mistake for real problems, and Lethal in the event the process can’t keep on.
Keep away from flooding your logs with excessive or irrelevant details. An excessive amount logging can obscure crucial messages and slow down your process. Deal with essential occasions, point out alterations, input/output values, and significant selection details with your code.
Format your log messages clearly and persistently. Consist of context, such as timestamps, request IDs, and performance names, so it’s simpler to trace issues in distributed systems or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
Throughout debugging, logs Enable you to track how variables evolve, what ailments are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re In particular precious in manufacturing environments wherever stepping by means of code isn’t probable.
Furthermore, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that help log rotation, filtering, and integration with checking dashboards.
Ultimately, smart logging is about stability and clarity. That has a nicely-imagined-out logging solution, you'll be able to decrease the time it's going to take to spot troubles, acquire deeper visibility into your apps, and Increase the General maintainability and dependability of your respective code.
Think Like a Detective
Debugging is not only a complex activity—it is a method of investigation. To effectively recognize and deal with bugs, builders should technique the procedure similar to a detective fixing a thriller. This mindset helps stop working advanced problems into manageable areas and abide by clues logically to uncover the basis bring about.
Get started by gathering evidence. Consider the signs or symptoms of the issue: mistake messages, incorrect output, or effectiveness troubles. Identical to a detective surveys against the law scene, obtain just as much relevant details as it is possible to devoid of leaping to conclusions. Use logs, exam circumstances, and consumer reviews to piece collectively a clear picture of what’s taking place.
Following, sort hypotheses. Check with you: What may be resulting in this actions? Have any variations a short while ago been made into the codebase? Has this difficulty happened in advance of beneath identical situation? The target should be to slender down options and establish likely culprits.
Then, examination your theories systematically. Make an effort to recreate the issue within a controlled ecosystem. For those who suspect a certain perform or component, isolate it and validate if the issue persists. Similar to a detective conducting interviews, check with your code queries and Allow the outcomes guide you closer to the reality.
Shell out close awareness to tiny details. Bugs generally hide within the the very least anticipated places—just like a lacking semicolon, an off-by-one particular error, or possibly a race situation. Be extensive and affected person, resisting the urge to patch the issue devoid of completely understanding it. Momentary fixes could disguise the true problem, only for it to resurface later.
And finally, hold notes on Everything you tried out and discovered. Equally as detectives log their investigations, documenting your debugging procedure can help save time for long term troubles and help Other folks understand your reasoning.
By wondering like a detective, developers can sharpen their analytical competencies, method troubles methodically, and come to be more effective at uncovering hidden difficulties in complex methods.
Write Exams
Producing checks is one of the most effective strategies to help your debugging skills and All round growth performance. Checks not merely enable capture bugs early but also serve as a safety net that gives you self-assurance when generating variations to your codebase. A nicely-analyzed software is much easier to debug mainly because it enables you to pinpoint specifically in which and when a difficulty happens.
Begin with unit exams, which give attention to unique capabilities or modules. These compact, isolated checks can immediately reveal whether or not a particular piece of logic is Doing work as predicted. Each time a check fails, you right away know exactly where to appear, considerably decreasing the time used debugging. Device assessments are Specifically helpful for catching regression bugs—problems that reappear after Formerly becoming fixed.
Future, combine integration exams and finish-to-end checks into your workflow. These support be certain that different parts of your application work jointly easily. They’re particularly practical for catching bugs that arise in complicated systems with many elements or services interacting. If a thing breaks, your exams can let you know which Element of the pipeline failed and under what ailments.
Creating checks also forces you to Imagine critically regarding your code. To test a attribute correctly, you require to be familiar with its inputs, predicted outputs, and edge instances. This standard of comprehending The natural way qualified prospects to raised code construction and less bugs.
When debugging an issue, composing a failing exam that reproduces the bug may be a powerful initial step. As soon as the test fails persistently, you can target correcting the bug and view your examination go when the issue is settled. This tactic ensures that a similar bug doesn’t return in the future.
In a nutshell, crafting tests turns debugging from a discouraging guessing game into a structured and predictable method—serving to you capture more bugs, more quickly plus much more reliably.
Get Breaks
When debugging a difficult challenge, it’s easy to become immersed in the issue—watching your display screen for several hours, seeking solution following Remedy. But Among the most underrated debugging applications is actually stepping absent. Getting breaks will help you reset your head, lower irritation, and infrequently see The difficulty from the new standpoint.
If you're far too near the code for much too extensive, cognitive fatigue sets in. You may begin overlooking apparent mistakes or misreading code that you simply wrote just hours earlier. In this point out, your Mind gets considerably less productive at difficulty-fixing. A short walk, a espresso split, as well as switching to a distinct activity for 10–quarter-hour can refresh your concentration. A lot of developers report discovering the foundation of a dilemma once they've taken time and energy to disconnect, allowing their subconscious function within the history.
Breaks also enable avert burnout, Specifically throughout longer debugging classes. Sitting in front of a display screen, mentally stuck, is not only unproductive but in addition draining. Stepping away means that you can return with renewed Electricity plus a clearer state of mind. You might quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded website you prior to.
For those who’re caught, a good guideline would be to set a timer—debug actively for forty five–60 minutes, then have a 5–10 moment split. Use that time to maneuver around, stretch, or do something unrelated to code. It could feel counterintuitive, Specifically less than restricted deadlines, but it really truly causes more quickly and more practical debugging In the end.
Briefly, using breaks will not be a sign of weak point—it’s a sensible method. It offers your Mind Place to breathe, improves your viewpoint, and allows you avoid the tunnel vision That always blocks your development. Debugging is actually a psychological puzzle, and relaxation is part of solving it.
Study From Each Bug
Each and every bug you face is a lot more than simply A short lived setback—It is a chance to improve as a developer. Regardless of whether it’s a syntax mistake, a logic flaw, or maybe a deep architectural issue, each one can educate you anything important if you take some time to mirror and examine what went Erroneous.
Commence by asking by yourself some critical thoughts once the bug is resolved: What caused it? Why did it go unnoticed? Could it happen to be caught earlier with far better procedures like unit testing, code evaluations, or logging? The solutions usually reveal blind places with your workflow or knowledge and make it easier to Make more robust coding behaviors transferring ahead.
Documenting bugs can be a superb routine. Preserve a developer journal or sustain a log where you note down bugs you’ve encountered, the way you solved them, and Anything you figured out. After some time, you’ll begin to see designs—recurring troubles or frequent blunders—that you could proactively avoid.
In workforce environments, sharing That which you've uncovered from a bug with your friends might be Specifically potent. Whether it’s via a Slack concept, a brief generate-up, or A fast expertise-sharing session, aiding others steer clear of the identical problem boosts workforce effectiveness and cultivates a stronger Mastering tradition.
Extra importantly, viewing bugs as lessons shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as necessary elements of your enhancement journey. In fact, several of the best builders are not the ones who write best code, but those who repeatedly learn from their problems.
In the end, Every single bug you fix adds a completely new layer for your ability established. So up coming time you squash a bug, have a instant to mirror—you’ll occur away a smarter, far more able developer due to it.
Summary
Improving upon your debugging abilities normally takes time, observe, and persistence — even so the payoff is large. It tends to make you a more successful, self-assured, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be better at Everything you do.